一. 简述
以可自定义的布局显示的数据项的有序集合。
@interface NSCollectionView : NSView
|
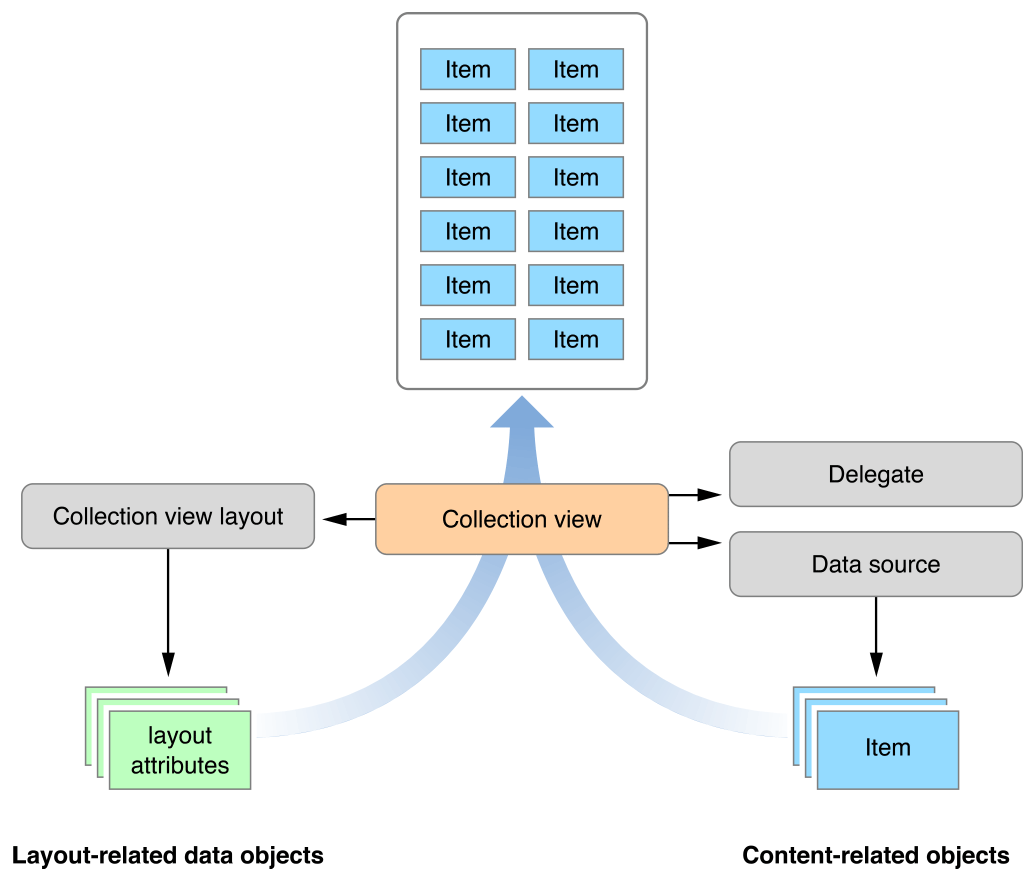
二. 官方属性方法
@property (nullable, weak) id<NSCollectionViewDataSource> dataSource API_AVAILABLE(macos(10.11)); @property (nullable, weak) id<NSCollectionViewPrefetching> prefetchDataSource API_AVAILABLE(macos(10.13));
@property (nullable, weak) id<NSCollectionViewDelegate> delegate;
@property (copy) NSArray<id> *content; @property (nullable, strong) NSView *backgroundView API_AVAILABLE(macos(10.11));
@property (null_resettable, copy) NSArray<NSColor *> *backgroundColors;
@property BOOL backgroundViewScrollsWithContent API_AVAILABLE(macos(10.12));
@property (nullable, strong) __kindof NSCollectionViewLayout *collectionViewLayout API_AVAILABLE(macos(10.11));
|
!!! 之后再补充 !!!
三. 示例
1. 效果图
要达到的效果:
- 显示左侧聊天列表
- Split滑动item内控件位置不变动
- 全屏下的显示
- 点击item调整选中色
如下图

2. 具体代码
2.1 创建NSCollectionView
此处为了方便使用Storyboard
创建

2.2 配置flowLayout(可以创建或者代理设置)
self.flowLayout = [[NSCollectionViewFlowLayout alloc] init]; self.flowLayout.itemSize = NSMakeSize(self.collectionView.frame.size.width, 60); self.flowLayout.minimumLineSpacing = 0; self.flowLayout.minimumInteritemSpacing = 0; self.collectionView.collectionViewLayout = self.flowLayout;
|
说明: 这里不使用Storyboard
或者代理配置flowLayout的原因是在滑动SplitView的时候要调整itemSize
,使用这种方式更方便设置itemSize
。
2.3 设置代理
@interface FSMessageVC () < NSSplitViewDelegate, NSCollectionViewDelegate, NSCollectionViewDataSource, NSCollectionViewDelegateFlowLayout >
self.collectionView.delegate = self; self.collectionView.dataSource = self;
|
2.4 设置CollectionView属性
self.collectionView.layer.backgroundColor = NSColor.whiteColor.CGColor; self.collectionView.wantsLayer = YES;
self.collectionView.selectable = YES;
|
2.5 注册自定义的Item
[self.collectionView registerNib:[[NSNib alloc] initWithNibNamed:@"FSChatCell" bundle:nil] forItemWithIdentifier:@"kChatItem"];
|
2.6 实现代理方法
- (NSInteger)numberOfSectionsInCollectionView:(NSCollectionView *)collectionView { return 1; }
- (NSInteger)collectionView:(NSCollectionView *)collectionView numberOfItemsInSection:(NSInteger)section { return self.dataSource.count; }
- (nonnull NSCollectionViewItem *)collectionView:(nonnull NSCollectionView *)collectionView itemForRepresentedObjectAtIndexPath:(nonnull NSIndexPath *)indexPath { FSChatCell *cell = (FSChatCell *)[collectionView makeItemWithIdentifier:@"kChatItem" forIndexPath:indexPath]; cell.name = self.dataSource[indexPath.item]; return cell; }
- (BOOL)commitEditingAndReturnError:(NSError *__autoreleasing _Nullable * _Nullable)error { return YES; }
- (void)encodeWithCoder:(nonnull NSCoder *)coder { }
|
2.7 Split滑动item内控件位置不变动
self.splitView.delegate = self;
- (CGFloat)splitView:(NSSplitView *)splitView constrainMinCoordinate:(CGFloat)proposedMinimumPosition ofSubviewAt:(NSInteger)dividerIndex { return 200; }
- (CGFloat)splitView:(NSSplitView *)splitView constrainMaxCoordinate:(CGFloat)proposedMaximumPosition ofSubviewAt:(NSInteger)dividerIndex { return 300; }
- (void)splitViewDidResizeSubviews:(NSNotification *)notification { self.flowLayout.itemSize = NSMakeSize(self.collectionView.frame.size.width, 60); }
|
2.8 全屏下的显示
添加全屏通知在通知的回调方法里调用 self.flowLayout.itemSize = NSMakeSize(self.collectionView.frame.size.width, 60);
,比较简单不再累述。
2.9 点击item调整选中背景色
有两种方式实现:
didSelectItemsAtIndexPaths
和didDeselectItemsAtIndexPaths
修改item背景色
- 在自定义item中重写
selected
方法
- (void)collectionView:(NSCollectionView *)collectionView didSelectItemsAtIndexPaths:(NSSet<NSIndexPath *> *)indexPaths { NSIndexPath *indexPath = indexPaths.allObjects.firstObject; FSChatCell *cell = (FSChatCell *)[collectionView itemAtIndexPath:indexPath]; cell.view.wantsLayer = YES; cell.view.layer.backgroundColor = NSColor.lightGrayColor.CGColor; }
- (void)collectionView:(NSCollectionView *)collectionView didDeselectItemsAtIndexPaths:(NSSet<NSIndexPath *> *)indexPaths { NSIndexPath *indexPath = indexPaths.allObjects.firstObject; FSChatCell *cell = (FSChatCell *)[collectionView itemAtIndexPath:indexPath]; cell.view.wantsLayer = YES; cell.view.layer.backgroundColor = NSColor.whiteColor.CGColor; }
- (void)viewDidLoad { [super viewDidLoad]; self.view.wantsLayer = YES; self.view.layer.backgroundColor = NSColor.whiteColor.CGColor; }
- (void)setSelected:(BOOL)selected { super.selected = selected; [self changeBgViewColor]; }
- (void)changeBgViewColor { if (self.selected) { if (self.highlightState == NSCollectionViewItemHighlightForSelection) { self.view.layer.backgroundColor = NSColor.lightGrayColor.CGColor; } else if (self.highlightState == NSCollectionViewItemHighlightNone || self.highlightState == NSCollectionViewItemHighlightForDeselection) { self.view.layer.backgroundColor = NSColor.whiteColor.CGColor; } } else { self.view.layer.backgroundColor = NSColor.whiteColor.CGColor; } }
|
2.10 FSChatCell.xib
说明:

- 连线时最好将控件连在创建的Objects-ChatCell上

2.11 添加头/尾
- (NSSize)collectionView:(NSCollectionView *)collectionView layout:(NSCollectionViewLayout *)collectionViewLayout referenceSizeForHeaderInSection:(NSInteger)section { return NSMakeSize(self.collectionView.frame.size.width, 40); }
- (NSView *)collectionView:(NSCollectionView *)collectionView viewForSupplementaryElementOfKind:(NSCollectionViewSupplementaryElementKind)kind atIndexPath:(NSIndexPath *)indexPath { FSChatHeaderView *headerView = [collectionView makeSupplementaryViewOfKind:NSCollectionElementKindSectionHeader withIdentifier:@"kChatHeader" forIndexPath:indexPath]; return headerView; }
|
Github Demo
说明: